Concept in All Programming Languages
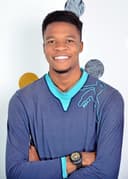
Benedict Anthony
October 15th 2024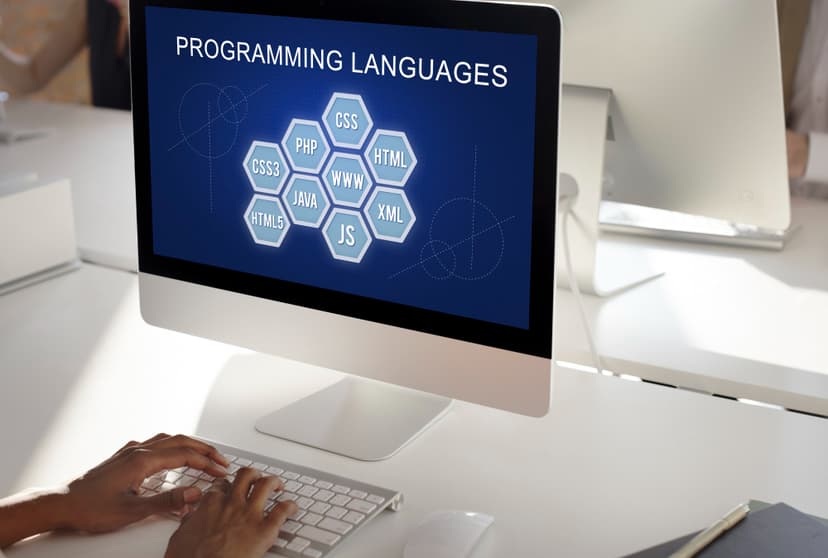
Introduction
Although programming languages are diverse, they all revolve around the same fundamental ideas. These ideas serve as the cornerstone on which programmers construct websites, software solutions, and applications. Gaining knowledge of these fundamental concepts improves your ability to switch between languages and provides you with a better understanding of how coding works in general. <br/> Let's examine the universal ideas that practically all programming languages share:
1. Variables and Data Types
Variables are the building blocks of any program. They store data values that can change over time or remain constant depending on how the program is structured. All programming languages offer support for variables, but the syntax for declaring them varies. The data types define what kind of data the variable can hold. Common Data Types: Integer: Whole numbers (e.g., int in C, int in Python), Float/Double: Decimal numbers (e.g., float in Python, double in Java), String: A sequence of characters (e.g., String in Java, str in Python), Boolean: A true or false value (e.g., bool in C++, boolean in JavaScript).
2. Control Flow Statements
Control flow determines the direction in which the program executes. Languages use control flow statements like conditionals and loops to dictate decisions and repeat actions. Common Control Flow Statements are Conditionals (if-else) to perform different actions based on conditions and Loops (for, while) to Repeat a block of code multiple times until a condition is met.
3. Functions/Methods
Functions (or methods in object-oriented languages) allow you to encapsulate blocks of code that perform a specific task. This modularity helps in breaking down complex problems and improves code reuse.
4. Object-Oriented Programming (OOP)
Object-oriented programming is a paradigm centered around the concept of “objects,” which can be thought of as real-world entities. Objects contain data (attributes) and behavior (methods). OOP is a fundamental concept in languages like Java, C++, Python, and C#. Key OOP Concepts includes Classes and Objects which defines a blueprint, and objects are instances of classes, Encapsulation which restrict access to certain details within an object, Inheritance, reusing code by inheriting from another class and Polymorphism, the ability to process objects differently based on their data type or class.
5. Error Handling
Handling errors gracefully is an important aspect of programming. Error handling involves detecting, catching, and managing exceptions that occur during execution.
6. Data Structures
Data structures are ways to organize and store data so that it can be accessed and modified efficiently. Almost all programming languages have built-in data structures, such as: Arrays/Lists: An ordered collection of elements (e.g., list in Python, array in Java), Dictionaries/Hash Maps: A collection of key-value pairs (e.g., dictionary in Python, HashMap in Java), Stacks and Queues: Structures that follow specific order rules (LIFO for stack, FIFO for queue), Trees and Graphs: Used in more complex applications like databases and network systems.
7. Libraries and Frameworks
Most languages come with built-in libraries or support third-party libraries and frameworks, allowing developers to speed up development by using pre-written code for common tasks. Libraries are collections of functions and objects, while frameworks are larger structures that provide a skeleton for applications.
8. Memory Management
Memory management refers to how a language handles the allocation and deallocation of memory during program execution. While some languages like C++ require manual memory management (using new and delete), others like Python and Java handle this automatically through garbage collection.
Conclusion
The concepts mentioned above serve as the backbone of all programming languages. By mastering these core ideas, you’ll find it easier to switch between languages and adapt to different programming paradigms. Whether you're building a mobile app in Swift or a web app in JavaScript, understanding these principles will make you a more versatile and proficient programmer.